-결과-
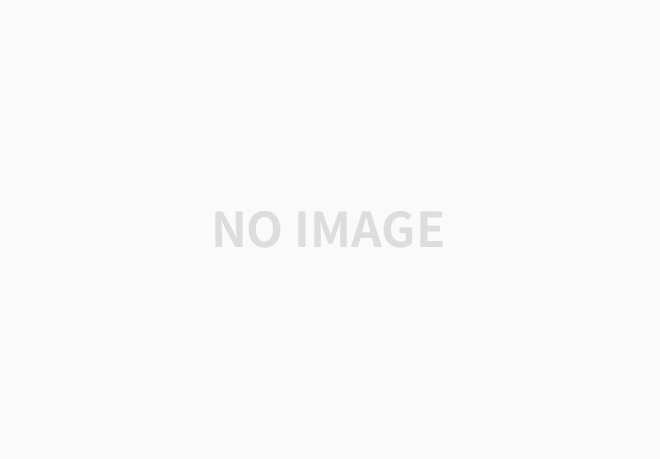
-구조-
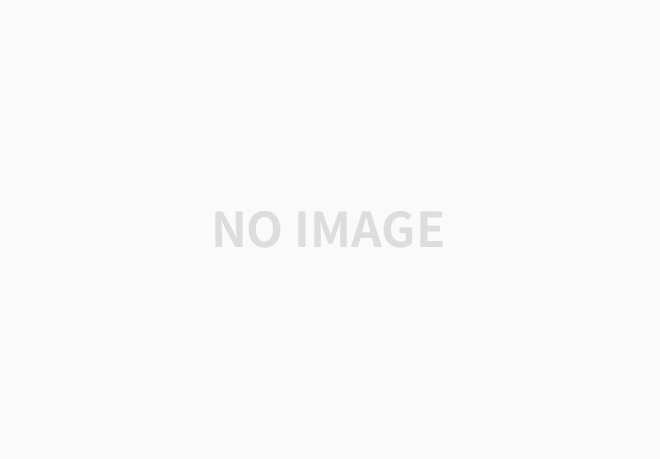
- 전체 코드 -
1) activity18_main.xml
<?xml version="1.0" encoding="utf-8"?>
<FrameLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="match_parent">
<LinearLayout
android:orientation="vertical"
android:background="#F0B6B6"
android:layout_width="match_parent"
android:layout_height="match_parent">
<TextView
android:textSize="30dp"
android:text="화면"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
</LinearLayout>
<LinearLayout
android:id="@+id/page"
android:orientation="vertical"
android:layout_width="200dp"
android:layout_height="match_parent"
android:layout_gravity="right"
android:background="#FF9F0E"
android:visibility="invisible">
<TextView
android:textSize="30dp"
android:text="sliding 옆영역"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
</LinearLayout>
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="열기"
android:layout_gravity="center_vertical|right"/>
<LinearLayout
android:id="@+id/page2"
android:orientation="vertical"
android:layout_width="match_parent"
android:layout_height="200dp"
android:layout_gravity="bottom"
android:background="#FF9F0E"
android:visibility="invisible">
<TextView
android:textSize="30dp"
android:text="sliding 아래영역"
android:layout_width="wrap_content"
android:layout_height="wrap_content" />
</LinearLayout>
<Button
android:id="@+id/button2"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="열기"
android:layout_gravity="center_horizontal|bottom"/>
</FrameLayout>
2) translate_left.xml
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android">
<!-- 어떻게 동작할 지를 적어준다
fromXDelta = "x의 시작 위치"
toXDelta = "x의 끝 위치"-->
<translate
android:fromXDelta="100%p"
android:toXDelta="0%p"
android:duration="500"
/>
</set>
3) translate_right.xml
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android">
<!-- 어떻게 동작할 지를 적어준다
fromXDelta = "x의 시작위치"
toXDelta = "x의 끝 위치"-->
<translate
android:fromXDelta="0%p"
android:toXDelta="100%p"
android:duration="500"
/>
</set>
4) translate_top.xml
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android">
<!-- 어떻게 동작할 지를 적어준다
fromYDelta = Y의 시작위치"
toYDelta = "Y의 끝위치"-->
<translate
android:fromYDelta="100%p"
android:toYDelta="0%p"
android:duration="500"
/>
</set>
5) translate_bottom.xml
<?xml version="1.0" encoding="utf-8"?>
<set xmlns:android="http://schemas.android.com/apk/res/android">
<!-- 어떻게 동작할 지를 적어준다
fromYDelta = Y의 시작위치"
toYDelta = "Y의 끝위치"-->
<translate
android:fromYDelta="0%p"
android:toYDelta="100%p"
android:duration="500"
/>
</set>
6) Activity18.java
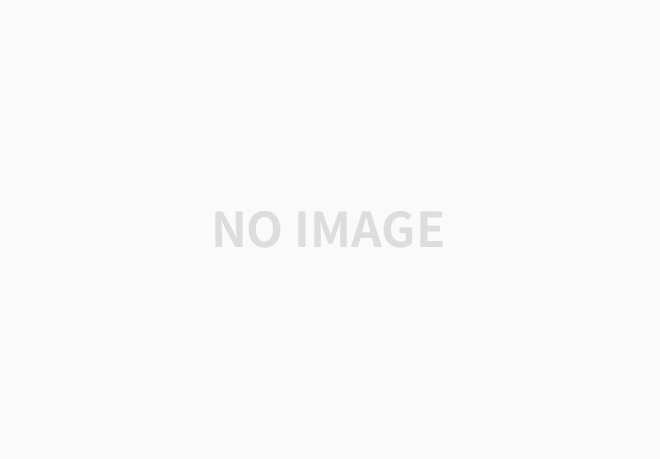
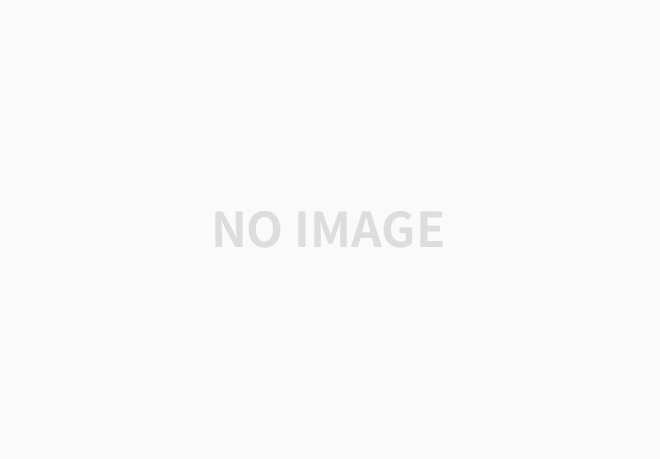
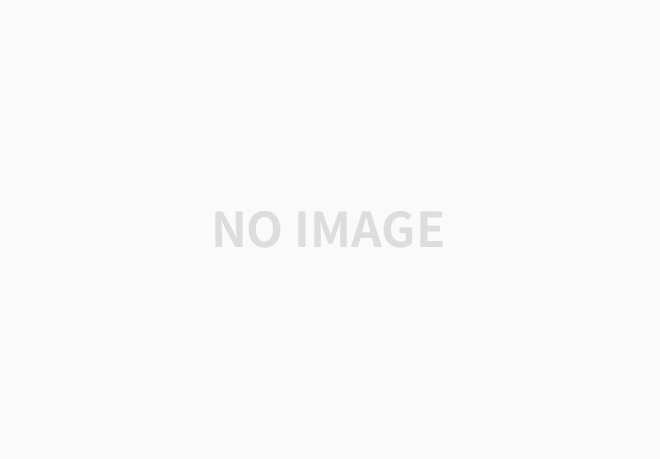
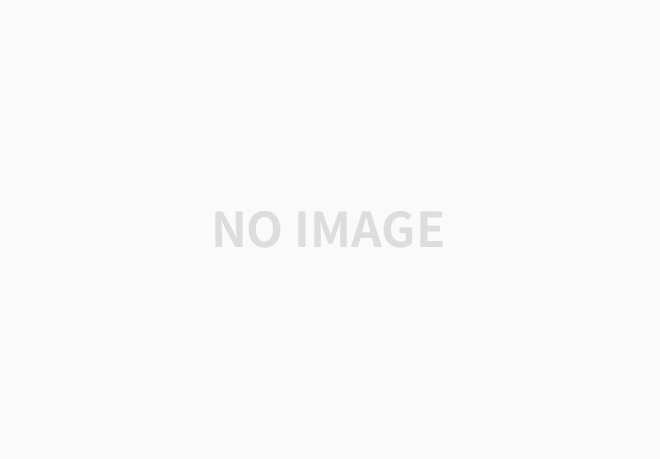
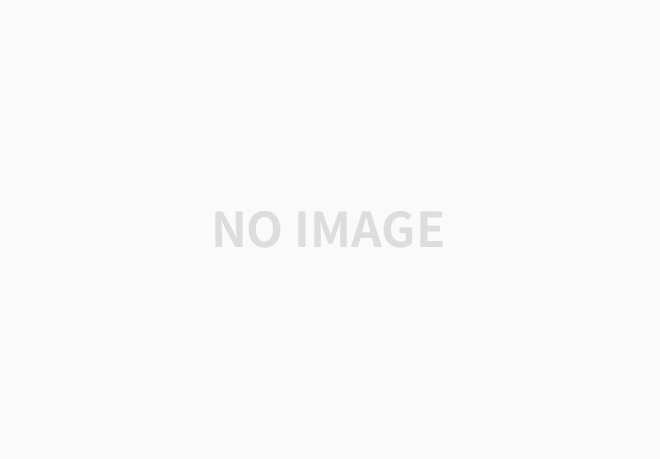
6) Activity18.java (똑같음)
package pagesliding18;
import android.os.Bundle;
import android.support.annotation.Nullable;
import android.support.v7.app.AppCompatActivity;
import android.view.View;
import android.view.animation.Animation;
import android.view.animation.AnimationUtils;
import android.widget.Button;
import android.widget.LinearLayout;
import com.example.noti.R;
public class Activity18 extends AppCompatActivity {
// 옆에서 슬라이드
LinearLayout page;
Animation translateLeft;
Animation translateRight;
Button button;
boolean isPageOpen =false;
//아래에서 슬라이드
LinearLayout page2;
Animation translateBottom;
Animation translateTop;
Button button2;
boolean isPageOpen2 =false;
@Override
protected void onCreate(@Nullable Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity18_main);
// 옆에서 슬라이드------------------------------------------------------------------
page = findViewById(R.id.page);
translateLeft = AnimationUtils.loadAnimation(this,R.anim.translate_left);
translateRight = AnimationUtils.loadAnimation(this,R.anim.translate_right);
SlidingAnimationListener listener = new SlidingAnimationListener();
translateLeft.setAnimationListener(listener);
translateRight.setAnimationListener(listener);
button=findViewById(R.id.button);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if(isPageOpen){
page.startAnimation(translateRight);
}else{
page.setVisibility(View.VISIBLE);
page.startAnimation(translateLeft);
}
}
});
// 옆에서 슬라이드------------------------------------------------------------------
//아래에서 슬라이드----------------------------------------------------------------
page2 = findViewById(R.id.page2);
translateBottom = AnimationUtils.loadAnimation(this,R.anim.translate_bottom);
translateTop = AnimationUtils.loadAnimation(this,R.anim.translate_top);
SlidingAnimationListener2 listener2 = new SlidingAnimationListener2();
translateBottom.setAnimationListener(listener2);
translateTop.setAnimationListener(listener2);
button2=findViewById(R.id.button2);
button2.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
if(isPageOpen2){
page2.startAnimation(translateBottom);
}else{
page2.setVisibility(View.VISIBLE);
page2.startAnimation(translateTop);
}
}
});
//아래에서 슬라이드----------------------------------------------------------------
}
class SlidingAnimationListener implements Animation.AnimationListener{
@Override
public void onAnimationStart(Animation animation) {
// 애니메이션 시작 될떄
}
@Override
public void onAnimationEnd(Animation animation) {
// 애니메이션 끝나는 시점
if(isPageOpen) {
page.setVisibility(View.INVISIBLE);
button.setText("열기");
isPageOpen=false;
}else{
button.setText("닫기");
isPageOpen =true;
}
}
@Override
public void onAnimationRepeat(Animation animation) {
// 반복되는 시점
}
}
class SlidingAnimationListener2 implements Animation.AnimationListener{
@Override
public void onAnimationStart(Animation animation) {
// 애니메이션 시작 될떄
}
@Override
public void onAnimationEnd(Animation animation) {
// 애니메이션 끝나는 시점
if(isPageOpen2) {
page2.setVisibility(View.INVISIBLE);
button2.setText("열기");
isPageOpen2=false;
}else{
button2.setText("닫기");
isPageOpen2=true;
}
}
@Override
public void onAnimationRepeat(Animation animation) {
// 반복되는 시점
}
}
}
'■ Android > Tip' 카테고리의 다른 글
[Android] 애니메이션 - 5.스플래시(Splash) 화면 (0) | 2020.03.08 |
---|---|
[Android] 애니메이션 - 4.속성 정리 (0) | 2020.03.07 |
[Android] 애니메이션 - 2.트윈 애니메이션 (0) | 2020.03.06 |
[Android] 애니메이션 - 1.스레드 애니메이션 (0) | 2020.03.06 |
[Android] 갤러리에서 이미지(사진) 가져오기 (0) | 2020.03.05 |